Authentication
The officially supported way to authenticate with the platform is to use OAuth 2.0, see OAuth Authentication Flow.
Add the user-specific Access Token that you obtain using said flow to every HTTP request to the API as a Bearer to an Authorization
header:
req, err := http.NewRequest("GET", "https://vizzlo.com/api/v1/user", nil) // <1>
req.Header.Set("Authorization", "Bearer " + token) // <2>
req.Header.Set("User-Agent", "ACME/1.0") // <3>
Code annotations
- Handle
err
appropiately. - Add the access token here.
- Add the name of your application as user agent to the request.
Additionally, there are three alternative ways to authenticate, which might help during the development phase of your client application:
- API Token configured by the user using the settings on the Vizzlo website. This token is to be send as part of an
Authorization
header asBearer
with every request. - Basic HTTP auth using user id and password.
- Session cookie based auth when logged into the website.
OAuth Authentication Flow
Vizzlo uses a standard OAuth 2.0 authentication flow. To use it, you need to register your application with Vizzlo. Please get in touch with help@vizzlo.com to do so. As part of the registration you will get a Client ID and a Client Secret.
OAuth2 configuration
Request Authorization URL
GET
https://vizzlo.com/oauth2/authorize
Access Token URL
POST
https://vizzlo.com/oauth2/token
Access Type
online
access is the only supported method at the moment.
Scopes
document
: Allows for handling user documents.
Example implementation
An example implementation in Go based on golang.org/x/oauth2 might look like this.
Code to direct user to Vizzlo so that they can grant your application access to the Vizzlo platform:
conf := &oauth2.Config{
ClientID: clientID, // <1>
ClientSecret: clientSecret, // <2>
Endpoint: oauth2.Endpoint{
AuthURL: "https://vizzlo.com/oauth2/authorize",
TokenURL: "https://vizzlo.com/oauth2/token",
},
RedirectURL: redirectURL, // <3>
Scopes: []string{"documents"},
}
sendUserHere := conf.AuthCodeURL(state, oauth2.AccessTypeOnline) // <4> <5>
Code annotations
- This is your application’s client ID.
- Add your client secret here.
- Add on of your configured redirect URLs here.
- The variable
state
should contians a random string which need to check later as a CSRF protection measure. - Redirect the user to the URL in
sendUserHere
.
Based on the above code, the user will be directed to Vizzlo (and, optionally, asked to log in or even create an account) and asked for permission to acces their Vizzlo account.
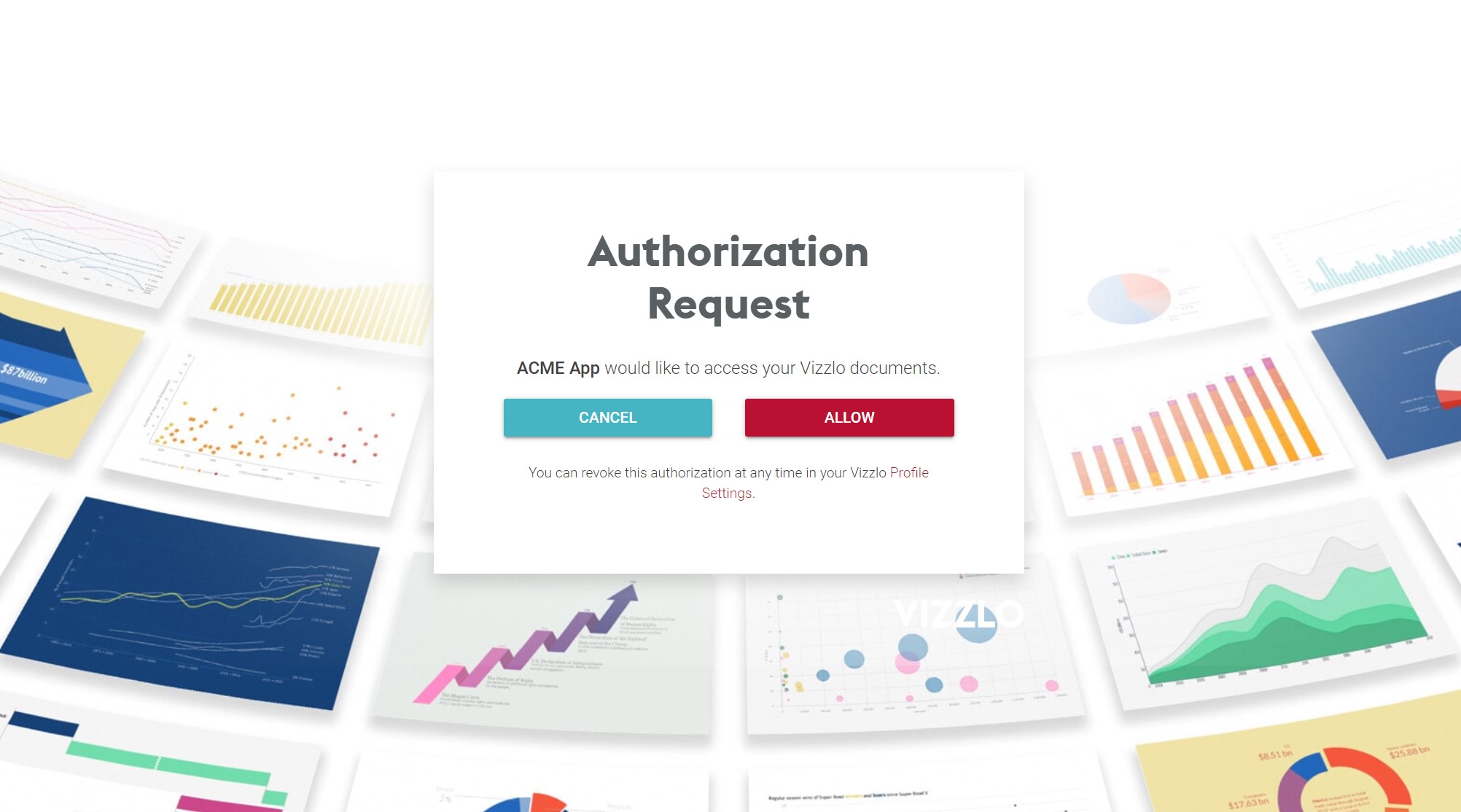
If the user authorizes the app, they will be directed back to your server (as per the redirectURL
specified) and your callback code should look roughtly like this:
tok, err := conf.Exchange(oauth2.NoContext, code) // <1> <2> <3>
accessToken := tok.AccessToken // <4>
Code annotations
- Here we assume that you successfully checked the
state
form value to exactly match the string you generated before redirecting the user to Vizzlo. - The variable
code
is gathered from a form value of the same name. - Handle the error
err
appropiately - Store
accessToken
in your database and use it for all subsequent API calls.